What's New in React 19? Key Differences from React 18 Explained
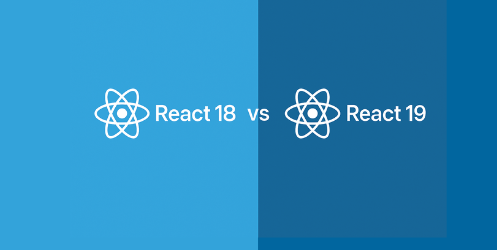
React.js
react19
ServerSideRendering
React 18 vs React 19
React 19 features
React upgrade guide
React is a popular JavaScript library for building dynamic and interactive user interfaces. Over the years, it has evolved significantly, adding new features, enhancing performance, and making it easier for developers to create powerful applications. In this article, we’ll compare React 18 and React 19 and explore the changes, updates, and improvements that come with each release.
1. Overview of React 18
React 18 brought several game-changing features designed to improve performance and offer more flexibility in building modern web applications. Some of the most notable features of React 18 included Concurrent Rendering, Automatic Batching, and Suspense for Data Fetching.
Key Features in React 18:
- Concurrent Rendering: This enables React to work on multiple tasks simultaneously, improving app performance, especially for complex UIs.
- Automatic Batching: React 18 automatically batches state updates to avoid unnecessary re-renders, optimizing rendering performance.
- Server-Side Rendering (SSR) with Suspense: React 18 made it easier to stream content from the server to the client.
- createRoot() API: React 18 introduced a new root API to allow apps to enable concurrent rendering.
Example of createRoot() in React 18:
1import ReactDOM from 'react-dom/client';
2import App from './App';
3
4// React 18 - new root API
5const root = ReactDOM.createRoot(document.getElementById('root'));
6root.render(<App />);
2. What’s New in React 19?
React 19 builds upon the foundation laid by React 18, introducing several important new features designed to help developers optimize their applications even further.
Key New Features in React 19:
- Server Components:
- React 19 fully supports React Server Components (RSC). This allows developers to create components that are rendered on the server and sent to the client, significantly improving load times by reducing the amount of JavaScript that needs to be sent to the browser.
1// ServerComponent.js
2export default function ServerComponent() {
3 return <div>This component is rendered on the server</div>;
4}
5
6// App.js
7import ServerComponent from './ServerComponent';
8export default function App() {
9 return (
10 <div>
11 <h1>Welcome to React 19</h1>
12 <ServerComponent />
13 </div>
14 );
15}
2. Smarter Hydration:
- React 19 introduces improved hydration logic, making it easier to handle SSR (Server-Side Rendering) mismatches. Hydration now works with partial updates to improve page load time.
Example:
1// In React 18, hydration was limited to the full tree
2ReactDOM.hydrate(<App />, document.getElementById('root'));
3
4// In React 19, partial hydration works smarter:
5ReactDOM.hydrate(<App />, document.getElementById('root'), {
6 onHydrated: () => console.log('Hydration complete')
7});
3. Developer Warnings and Enhanced Debugging:
- React 19 now provides more contextually relevant warnings during development, making it easier for developers to identify issues with rendering, state management, and props handling.
Example:
1// React 19 will show more specific warnings if something breaks:
2if (someValue === undefined) {
3 console.warn('The value is undefined, check your props');
4}
4. Performance Improvements with Suspense:
- React 19 improves the use of Suspense in concurrent rendering. This helps in building apps that load and render faster by managing components’ loading states.
Example of Suspense:
1import React, { Suspense } from 'react';
2
3const LazyComponent = React.lazy(() => import('./LazyComponent'));
4
5function App() {
6 return (
7 <Suspense fallback={<div>Loading...</div>}>
8 <LazyComponent />
9 </Suspense>
10 );
11}
3. Performance Improvements
React 19 introduces several performance optimizations aimed at improving the overall speed of web applications:
- Partial Hydration: React 19 hydrates only the parts of the UI that need it, reducing unnecessary rendering, which leads to faster page loads.
- Smart Scheduling: React 19 schedules tasks more efficiently, using the Idle Time API and requestIdleCallback to perform less critical updates during idle times.
- Concurrent Rendering Optimization: Tasks are given priorities, so React can render high-priority tasks first and defer less important tasks.
Example of Optimized Rendering:
1// React 19 - Prioritizing high-priority tasks
2startTransition(() => {
3 // Low-priority state update
4 setLowPriorityState(newState);
5});
6
7setHighPriorityState(highPriorityData); // High-priority update
4. Key Feature Comparisons: React 18 vs React 19
Feature | React 18 | React 19 |
---|---|---|
Concurrent Rendering | ✅ Introduced | ✅ Improved |
Server Components | 🚫 Experimental | ✅ Fully supported |
Suspense Improvements | ✅ Basic support | ✅ Advanced boundaries |
Hydration | ⚠️ Basic hydration logic | ✅ Smarter, partial hydration |
useFormStatus / useFormState | 🚫 Not available | ✅ Available |
TypeScript Support | ✅ Good | ✅ Enhanced |
Compiler Optimizations | 🚫 Minimal | ✅ Smart component analysis |
Developer Warnings | ⚠️ Limited | ✅ Detailed and contextual |
5. Breaking Changes in React 19
While React 19 is mostly backward compatible with React 18, there are a few breaking changes:
- Deprecated APIs: React 19 drops support for older APIs like ReactDOM.render(). Instead, you must use ReactDOM.createRoot() for concurrent rendering.
- Suspense Changes: React 19 optimizes Suspense, meaning some of your existing Suspense boundaries might behave differently.
- New Error Boundaries: More granular error boundaries are now required in certain asynchronous rendering situations.
1// React 19 warns if you're still using the deprecated API:
2ReactDOM.render(<App />, document.getElementById('root')); // Deprecated
6. Should You Upgrade to React 19?
If your app is using React 18 and you want to take advantage of new features like Server Components, smarter hydration, or advanced Suspense handling, React 19 is a great choice. However, you should ensure that your app is ready for these changes.
When to upgrade:
- When your app requires Server-Side Rendering (SSR) or Server Components.
- When you're building large-scale apps that need better performance optimizations.
- When you want access to better developer warnings and debugging tools.
7. Concurrent Mode Improvements
In React 18, Concurrent Mode was introduced as an experimental feature, enabling apps to be more responsive by rendering multiple tasks concurrently without blocking the main thread. React 19 refines this feature to provide better stability and more control over concurrent rendering, making it easier for developers to implement high-performance applications.
Key Enhancements in Concurrent Mode:
- Improved Task Prioritization: React 19 introduces smarter prioritization of tasks so that high-priority updates (e.g., user interactions) are rendered faster while low-priority tasks (like data fetching) can be deferred.
Example of a concurrent update:
1// React 19 makes it easier to prioritize tasks:
2startTransition(() => {
3 // Low-priority task (e.g., background update)
4 setLowPriorityData(newData);
5});
6
7setHighPriorityData(highPriorityData); // High-priority update
8. Automatic Batching Enhancements
React 18 introduced automatic batching, where multiple state updates within a single event are batched together to avoid unnecessary re-renders. In React 19, this feature has been enhanced to work in asynchronous events, such as setTimeout or promises, ensuring even more efficient re-renders.
Automatic Batching in React 19:
React 19 now batches state updates automatically even if they're inside asynchronous operations like setTimeout or API calls. This results in fewer re-renders and better performance, especially in large applications.
Example:
1setTimeout(() => {
2 // React 19 will batch both updates together
3 setState1(newState1);
4 setState2(newState2);
5}, 1000);
9. React Suspense for Data Fetching
React 18 introduced Suspense as a way to handle asynchronous data fetching in a declarative manner. React 19 improves this feature by making it more powerful, with suspense boundaries now working seamlessly for data fetching in both client-side and server-side rendering scenarios.
How Suspense Has Evolved:
In React 18, Suspense was mainly used for code splitting and lazy loading components. In React 19, it is extended to support data fetching by allowing the app to suspend its rendering while waiting for an API response or data to load.
Example:
1const DataFetchingComponent = React.lazy(() => import('./DataFetchingComponent'));
2
3function App() {
4 return (
5 <Suspense fallback={<div>Loading data...</div>}>
6 <DataFetchingComponent />
7 </Suspense>
8 );
9}
10. Server-Side Rendering (SSR) and Streaming
With React 18, Server-Side Rendering (SSR) introduced Suspense for SSR, which allows for rendering HTML on the server side and streaming it to the client. React 19 takes this a step further by introducing React Streaming — which allows chunks of the application to be streamed to the client as they are generated, rather than waiting for the entire page to load.
Streaming in React 19:
- Partial Rendering: React 19 allows applications to stream partial content to users, improving perceived performance.
- Optimized SSR: It supports progressive hydration, where the client can start interacting with the UI before the entire JavaScript bundle is fully loaded.
Example of Streaming in SSR:
1// Streaming SSR in React 19 with a streaming server
2const app = express();
3app.use(reactDomServer.renderToNodeStream(<App />));
11. React Server Components (RSC)
One of the most anticipated features of React 19 is React Server Components (RSC). Server components are designed to allow you to offload rendering work to the server, while still delivering a fully interactive experience to users.
Key Features of RSC:
- Zero-Bundle-Size for Server Components: Server components don’t add any JavaScript to the bundle, as they are rendered on the server and sent to the client.
- Hybrid Rendering: Developers can now combine client and server-side components in the same component tree, improving the efficiency of rendering.
Example of a Server Component:
1// This component is rendered on the server
2export function ServerComponent() {
3 return <div>This content was rendered on the server.</div>;
4}
5
6// Client-side component that interacts with the server-rendered content
7export function ClientComponent() {
8 return <div>This content is client-rendered.</div>;
9}
12. Improved TypeScript Support
React 19 brings enhanced TypeScript definitions for better type inference and integration. This is an important upgrade, especially as React has become widely used in TypeScript projects.
Key Improvements:
- More Precise Types: React 19 improves type safety with better typings for hooks like useState and useEffect.
- Better Type Inference: React 19 also brings better support for TypeScript’s infer functionality, which makes working with complex state easier and less error-prone.
Example of TypeScript in React 19:
1const [count, setCount] = useState<number>(0); // React 19 provides more accurate types
2
3useEffect(() => {
4 console.log(`Count has changed to ${count}`);
5}, [count]);
6
13. JSX Transform Improvements
React 19 also introduces an improved JSX transform, which automatically optimizes the compilation of JSX. This means that the transformation of JSX syntax into JavaScript is more efficient, reducing the need for React-specific imports and improving bundle sizes.
JSX Transform Example:
javascriptCopyEdit
1// In React 18, we need to import React explicitly:
2import React from 'react';
3
4const App = () => {
5 return <div>Hello World</div>;
6};
7
8// In React 19, React import is not necessary anymore:
9const App = () => {
10 return <div>Hello World</div>;
11};
14. New Concurrent Rendering Features for React 19
While React 18 introduced Concurrent Rendering, React 19 further improves this feature with a new scheduling algorithm. This allows React to interrupt rendering when higher-priority tasks need to be performed, providing a more fluid experience for users.
- Prioritizing User Input: React 19 makes it easier to ensure that user interactions (such as clicks and typing) are always processed immediately.
- Defer Lower-Priority Updates: Non-urgent UI updates (like background tasks) can be deferred to improve perceived performance.
Example:
1startTransition(() => {
2 setBackgroundData(fetchedData); // Non-urgent update
3});
15. Enhanced Error Boundaries in React 19
Error boundaries were introduced in React 16 to catch JavaScript errors anywhere in a component tree and log them. React 19 takes this feature further by allowing granular error boundaries within concurrent rendering. This means that errors in certain components don’t bring down the entire app.
Example of an Error Boundary:
1class MyErrorBoundary extends React.Component {
2 state = { hasError: false };
3
4 static getDerivedStateFromError(error) {
5 return { hasError: true };
6 }
7
8 componentDidCatch(error, info) {
9 console.error('Error caught by boundary:', error, info);
10 }
11
12 render() {
13 if (this.state.hasError) {
14 return <h1>Something went wrong.</h1>;
15 }
16 return this.props.children;
17 }
18}
19
20<MyErrorBoundary>
21 <App />
22</MyErrorBoundary>
17. Updated DevTools in React 19
React 19 introduces improved React DevTools for both the browser and standalone versions, making it easier to inspect component trees and diagnose performance bottlenecks.
DevTools Improvements:
- Better Support for Concurrent Mode: Developers can now see which components are being rendered concurrently.
- Profiling Tools: React 19 provides a profiling tool that helps you track the performance of individual components.
Conclusion
React 19 introduces a wide range of improvements, optimizations, and new features compared to React 18. From Concurrent Rendering, Server Components, and Suspense for data fetching, to smarter hydration and better TypeScript support, React 19 provides developers with powerful tools to build fast and scalable applications.
By leveraging these features, you can improve your app’s performance, optimize user experience, and streamline the development process. As always, testing is crucial when upgrading to a new version, especially with React’s cutting-edge features.