Next.js Performance Mastery: Caching, Lazy Loading, and Image Optimization Explained
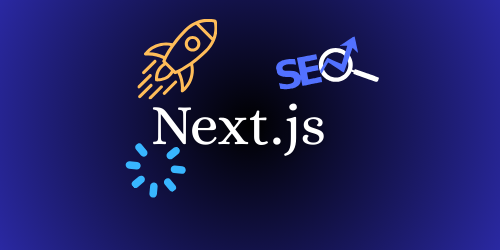
NextJS
LazyLoading
ImageOptimization
NextJSTutorial
ReactJS
CodeSplitting
WebOptimization
FrontendDevelopment
SiteSpeed
Discover how to supercharge your Next.js applications with proven performance techniques. Learn to lazy load heavy components, optimize images using next/image, implement smart caching with ISR and SWR and leverage code splitting and prefetching for lightning-fast navigation.
📝 Introduction
Performance is not optional anymore.
Google, users, and SEO all demand fast, smooth, and optimized websites. Luckily, Next.js offers everything you need to:
- Load images only when needed
- Cache pages and data intelligently
- Minimize bundle size with code splitting
- Make navigation super fast with prefetching
Let’s break this down step-by-step with code, real use cases, and visual examples.
1. Lazy Loading Components in Next.js
🧠 What is it?
Lazy loading means loading something only when it’s needed. Instead of loading heavy components (charts, modals, third-party widgets) right away, we delay their loading.
This reduces the initial JavaScript bundle size, making your page load faster.
✅ Step-by-step Code Example
🧩 Step 1: Create a heavy component
1// components/HeavyChart.tsx
2export default function HeavyChart() {
3 return <div>📊 I am a heavy chart loaded only when needed!</div>;
4}
🚀 Step 2: Dynamically load it using next/dynamic
1// pages/index.tsx
2import dynamic from 'next/dynamic';
3import { useState } from 'react';
4
5// Lazy-load the component
6const HeavyChart = dynamic(() => import('../components/HeavyChart'), {
7 loading: () => <p>Loading chart...</p>,
8 ssr: false, // disables server-side rendering for this component
9});
10
11export default function Home() {
12 const [showChart, setShowChart] = useState(false);
13
14 return (
15 <div>
16 <h1>Welcome to Performance-Optimized App 🚀</h1>
17 <button onClick={() => setShowChart(true)}>Load Chart</button>
18 {showChart && <HeavyChart />}
19 </div>
20 );
21}
22
🔍 Result:
- Initial page loads fast without loading the chart.
- Chart loads only when the button is clicked.
2. Image Optimization with next/image
🧠 What is it?
Images are often the largest resources on a page. Poorly optimized images = slow pages.
Next.js provides an <Image /> component that:
- Automatically lazy-loads images
- Converts them to modern formats (like WebP)
- Serves responsive sizes
- Includes blur placeholders
✅ Step-by-step Code Example
Basic usage:
1import Image from 'next/image';
2
3export default function HeroImage() {
4 return (
5 <Image
6 src="/images/hero.jpg"
7 alt="Hero"
8 width={1200}
9 height={600}
10 priority // loads immediately for above-the-fold images
11 />
12 );
13}
Blur placeholder (smooth loading experience)
1<Image
2 src="/images/product.jpg"
3 alt="Product"
4 width={600}
5 height={400}
6 placeholder="blur"
7 blurDataURL="/images/blurred.jpg"
8/>
3. Caching Strategies in Next.js
🧠 What is it?
Caching stores data so it's not reloaded from scratch every time. This applies to:
- Pages (using ISR – Incremental Static Regeneration)
- API responses (with SWR)
- Headers (for static assets)
✅ a. ISR (Incremental Static Regeneration)
Update static pages without full redeployment.
1// pages/products/[id].tsx
2export async function getStaticPaths() {
3 const res = await fetch('https://api.example.com/products');
4 const products = await res.json();
5
6 return {
7 paths: products.map((p) => ({ params: { id: p.id.toString() } })),
8 fallback: 'blocking',
9 };
10}
11
12export async function getStaticProps({ params }) {
13 const res = await fetch(`https://api.example.com/products/${params.id}`);
14 const product = await res.json();
15
16 return {
17 props: { product },
18 revalidate: 60, // Page will be regenerated every 60 seconds
19 };
20}
✅ b. Client-Side Caching with SWR
Install first:
1npm install swr
Then use:
1import useSWR from 'swr';
2
3const fetcher = (url) => fetch(url).then((res) => res.json());
4
5export default function ProductList() {
6 const { data, error } = useSWR('/api/products', fetcher);
7
8 if (error) return <p>❌ Failed to load products</p>;
9 if (!data) return <p>⏳ Loading...</p>;
10
11 return (
12 <ul>
13 {data.map((product) => (
14 <li key={product.id}>{product.name}</li>
15 ))}
16 </ul>
17 );
18}
✅ c. Set Cache Headers (Edge Middleware)
In Next.js 14, you can control headers with middleware:
1// middleware.ts
2import { NextResponse } from 'next/server';
3
4export function middleware(request) {
5 const response = NextResponse.next();
6 response.headers.set('Cache-Control', 'public, max-age=3600');
7 return response;
8}
4. Code Splitting & Prefetching
🧠 What is it?
Next.js automatically splits your code by route and preloads pages for fast navigation.
✅ Link prefetching (default behavior)
1import Link from 'next/link';
2
3export default function Nav() {
4 return (
5 <nav>
6 <Link href="/about">About Us</Link>
7 </nav>
8 );
9}
🧠 How it helps:
- When a link becomes visible in the viewport, Next.js preloads the JavaScript bundle.
- Clicking is instant — like a native app.
🧪 Bonus: Monitor & Audit Your App
✅ Use Lighthouse (in Chrome DevTools)
Steps:
- Open your app
- Press F12 → Go to “Lighthouse” tab
- Click “Generate Report”
Check:
- First Contentful Paint (FCP)
- Largest Contentful Paint (LCP)
- Time to Interactive (TTI)
5. SEO in Next.js
1. Use <Head> from next/head
To add meta tags like title, description, keywords, etc., use the Head component.
1// pages/index.tsx
2import Head from 'next/head';
3
4export default function Home() {
5 return (
6 <>
7 <Head>
8 <title>My Blog | Learn Next.js SEO</title>
9 <meta name="description" content="Learn how to optimize your Next.js website for SEO with basic and advanced techniques." />
10 <meta name="keywords" content="Next.js, SEO, React, Web Performance" />
11 <meta name="author" content="Your Name" />
12 </Head>
13
14 <main>
15 <h1>Welcome to My SEO Blog</h1>
16 </main>
17 </>
18 );
19}
2. Page Titles & Descriptions Per Page
Each page should have its own unique <title> and <meta name="description">.
📈 Intermediate SEO Techniques
3. Use next/image for Image Optimization
Next.js automatically optimizes images for SEO and performance. Alt text improves accessibility and SEO.
1import Image from 'next/image';
2
3<Image
4 src="/blog-cover.jpg"
5 alt="A beautiful cover image for SEO tips"
6 width={800}
7 height={400}
8 priority
9/>
✅ Final Tips for 2025
Tip | Why It Matters |
---|---|
Use <Image /> instead of <img> | For automatic lazy loading and responsiveness |
Lazy-load components you don’t need initially | Keeps bundle small and fast |
Lazy-load components you don’t need initially | Keeps bundle small and fast |
Enable ISR for dynamic content | Keeps static site fast but fresh |
Use SWR for client-side caching | Reduces API load and re-fetches |
Minimize third-party scripts | They block rendering |
<Head> with title/desc | Meta info for search engines |
next/image | Fast images, better UX & SEO |