Mastering SEO in Next.js 15 (2025): The Complete Guide from Basics to Advanced
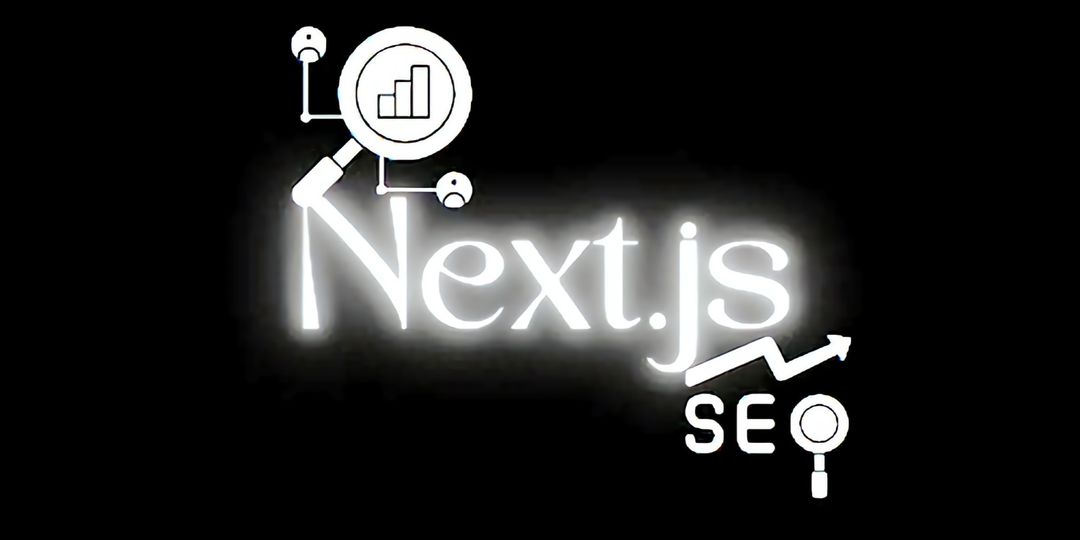
NextJS15
NextJSSEO
SEO2025
ReactJS
Metadata
OpenGraph
JSONLD
StaticSiteGeneration
With the release of Next.js 15, Vercel has taken performance, routing, and SEO capabilities to a new level. SEO is still a major player in how websites are discovered and ranked in search engines like Google. The latest updates to Next.js — including the App Router, partial pre-rendering, enhanced metadata API, and built-in image/CDN optimizations — are game changers for SEO.
✍️ Introduction
With the release of Next.js 15, Vercel has taken performance, routing, and SEO capabilities to a new level. SEO is still a major player in how websites are discovered and ranked in search engines like Google. The latest updates to Next.js — including the App Router, partial pre-rendering, enhanced metadata API, and built-in image/CDN optimizations — are game changers for SEO.
This 2025 guide will walk you through everything you need to know about SEO in Next.js 15 — starting from the basics and ending with advanced strategies used in production-grade apps.
🧠 What’s New in Next.js 15 for SEO?
Here are key SEO-related features in Next.js 15:
Feature | SEO Benefit |
---|---|
App Router (Stable) | Structured routing, layouts, metadata support |
New metadata API | Dynamic and static SEO metadata without using next/head |
Partial Prerendering (Beta) | Faster page loads with SEO-friendly hybrid rendering |
Enhanced Image Optimization | Better LCP and CLS scores |
Improved robots.txt and sitemap handling | Cleaner setup with tools like next-sitemap |
File-based route groups and intercepting routes | Better UX, breadcrumb logic, and crawl optimization |
✅ Basic SEO in Next.js 15 (2025)
1. Define SEO Metadata Using metadata API (New!)
No need to use <Head> manually in the App Router. Use the metadata export in your page.tsx or layout.tsx.
1// app/blog/page.tsx
2
3export const metadata = {
4 title: "Top 10 Coffee Shops in NYC | MyCoffeeBlog",
5 description: "Explore top-rated coffee shops in New York City with reviews, photos, and directions.",
6 robots: "index, follow",
7 openGraph: {
8 title: "Top 10 Coffee Shops in NYC",
9 description: "Handpicked NYC cafés for true coffee lovers.",
10 url: "https://yourdomain.com/blog",
11 images: [
12 {
13 url: "/images/coffee.jpg",
14 width: 800,
15 height: 600,
16 },
17 ],
18 },
19};
20
21export default function BlogPage() {
22 return (
23 <main>
24 <h1>Top Coffee Shops in NYC</h1>
25 <p>Welcome to the ultimate coffee guide!</p>
26 </main>
27 );
28}
✅ Cleaner
✅ Static and dynamic metadata support
✅ Great for social media previews
2. Use Semantic and Accessible HTML
Always use proper HTML structure — this helps search engines understand your content better.
tsxCopyEdit
1<main>
2 <article>
3 <header>
4 <h1>Top Coffee Shops in Brooklyn</h1>
5 <time dateTime="2025-05-01">May 1, 2025</time>
6 </header>
7 <p>Our updated guide to the best Brooklyn cafés.</p>
8 </article>
9</main>
3. Use Clean URLs with App Router
Next.js 15’s App Router continues file-based routing with layouts and route groups.
1/app
2 /blog
3 page.tsx → /blog
4 /[slug]
5 page.tsx → /blog/best-coffee-brooklyn
👍 Clean URLs are essential for keyword optimization.
⚙️ Intermediate SEO in Next.js 15
4. Generate a Sitemap and robots.txt Automatically
Use next-sitemap (compatible with App Router):
1npm install next-sitemap
next-sitemap.config.js:
1module.exports = {
2 siteUrl: 'https://yourdomain.com',
3 generateRobotsTxt: true,
4 sitemapSize: 7000,
5};
Add to package.json:
1"scripts": {
2 "postbuild": "next-sitemap"
3}
This creates:
- public/sitemap.xml
- public/robots.txt
5. Add Canonical URLs
Avoid duplicate content issues.
1export const metadata = {
2 title: "Best Coffee in NYC",
3 alternates: {
4 canonical: "https://yourdomain.com/blog/best-coffee-nyc",
5 },
6};
6. Optimize Performance for Core Web Vitals
Next.js 15 improves TTFB and LCP with:
- Streaming layouts
- Partial prerendering
- Edge middleware
✅ Use next/image:
1import Image from "next/image";
2
3<Image
4 src="/images/coffee.jpg"
5 alt="NYC coffee shop"
6 width={800}
7 height={500}
8 priority
9/>
✅ Lazy load non-critical components
✅ Use static rendering (generateStaticParams + generateMetadata) when possible
🔬 Advanced SEO Techniques in Next.js 15
7. Dynamic Metadata with generateMetadata
Example: blog pages with dynamic meta tags from a CMS or API.
1// app/blog/[slug]/page.tsx
2import { getPostData } from '@/lib/api';
3
4export async function generateMetadata({ params }) {
5 const post = await getPostData(params.slug);
6 return {
7 title: post.title,
8 description: post.excerpt,
9 openGraph: {
10 title: post.title,
11 description: post.excerpt,
12 url: `https://yourdomain.com/blog/${params.slug}`,
13 },
14 };
15}
8. Structured Data (JSON-LD)
Improves visibility in Google rich results.
1<Script id="ld-json" type="application/ld+json">
2 {JSON.stringify({
3 "@context": "https://schema.org",
4 "@type": "Article",
5 "headline": "Top 10 Coffee Shops in NYC",
6 "author": {
7 "@type": "Person",
8 "name": "John Doe"
9 },
10 "datePublished": "2025-05-01",
11 "publisher": {
12 "@type": "Organization",
13 "name": "CoffeeBlog Inc."
14 }
15 })}
16</Script>
9. Localization SEO with i18n Routing
1// next.config.js
2module.exports = {
3 i18n: {
4 locales: ['en', 'es', 'fr'],
5 defaultLocale: 'en',
6 },
7};
Now your pages are served at:
- /en/blog
- /es/blog
- /fr/blog
Add hreflang for Google to properly index regional versions.
10. Breadcrumbs, Schema & UX Signals
Use structured breadcrumbs and good UX (loading fast, clean structure).
1<Script id="breadcrumb-schema" type="application/ld+json">
2 {JSON.stringify({
3 "@context": "https://schema.org",
4 "@type": "BreadcrumbList",
5 "itemListElement": [
6 {
7 "@type": "ListItem",
8 "position": 1,
9 "name": "Blog",
10 "item": "https://yourdomain.com/blog"
11 },
12 {
13 "@type": "ListItem",
14 "position": 2,
15 "name": "Best Coffee in NYC",
16 "item": "https://yourdomain.com/blog/best-coffee-nyc"
17 }
18 ]
19 })}
20</Script>
📊 Recommended Tools for 2025
Tool | Purpose |
---|---|
Google Search Console | Track indexing and ranking |
Ahrefs / Semrush / Ubersuggest | Keyword & backlink research |
next-sitemap | SEO sitemap and robots.txt generation |
Lighthouse CI | Measure Core Web Vitals |
Vercel Analytics | Performance & engagement tracking |
✅ Final SEO Checklist for 2025
- Use metadata API for title & description
- Add Open Graph & Twitter meta for social previews
- Use generateMetadata for dynamic pages
- Add canonical URLs to avoid duplicate content
- Optimize images with next/image
- Generate sitemap and robots.txt
- Add JSON-LD structured data
- Ensure fast load time, good Core Web Vitals
- Use semantic HTML and good UX
🎯 Conclusion
Next.js 15 in 2025 is the most SEO-friendly version yet, thanks to the App Router, dynamic metadata, partial rendering, and powerful static features.
By following the strategies in this guide — from setting up metadata to structured data and performance optimization — you can greatly improve your search visibility, user engagement, and traffic.