How to Integrate Sanity CMS with Next.js for a Powerful Headless Experience
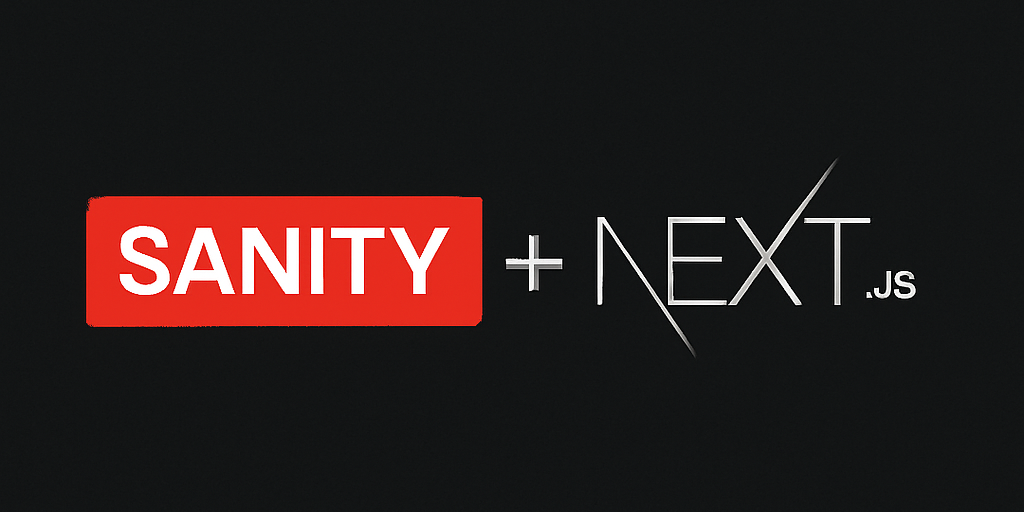
Next.js
sanity
Handless CMS
serverless
In the modern web development world, content is king. But managing it efficiently is just as important. That’s where headless CMSs like Sanity.io and frameworks like Next.js come in. This blog will walk you through integrating Sanity with Next.js — creating a scalable, SEO-friendly, and developer-friendly application.
🏗️ What Is Sanity?
Sanity.io is a powerful headless CMS that offers real-time collaboration, customizable content structures, and excellent developer tools. It’s ideal for developers who want to control both the content model and the front end.
⚙️ Why Use Sanity with Next.js?
- 🔄 Real-time data editing and preview
- ⚡ Super-fast with Static Site Generation (SSG) and Incremental Static Regeneration (ISR)
- 💾 Structured content with GROQ queries
- 📦 Portable text for rich content rendering
- 🌐 Headless — perfect for JAMstack apps
🧰 Prerequisites
Make sure you have the following before you begin:
- Node.js and npm/yarn installed
- Next.js project setup
- Basic knowledge of React and API calls
📦 Step-by-Step: Sanity + Next.js Integration
1. ✅ Set up a Sanity Studio
bashCopyEditnpm create sanity@latest
1npm create sanity@latest
- Choose a project name.
- Select the Blog (schema) template
- Deploy using Sanity’s CLI
1sanity deploy
bashCopyEditsanity deploy
💡 You’ll get a Sanity Studio at a hosted URL.
2. ✅ Define Your Schema (e.g., post.js)
1export default {
2 name: 'post',
3 type: 'document',
4 title: 'Post',
5 fields: [
6 { name: 'title', type: 'string' },
7 { name: 'slug', type: 'slug', options: { source: 'title' } },
8 { name: 'body', type: 'blockContent' },
9 { name: 'mainImage', type: 'image' },
10 ]
11}
12
3. ✅ Connect Sanity in Next.js
Install the Sanity client:
1npm install @sanity/client @portabletext/react
Create a client file in lib/sanity.js:
1import { createClient } from '@sanity/client'
2
3export const client = createClient({
4 projectId: 'your_project_id',
5 dataset: 'production',
6 apiVersion: '2023-01-01',
7 useCdn: true
8})
4. ✅ Fetch Data Using GROQ
GROQ (Graph-Relational Object Queries) is Sanity's query language:
1const query = `*[_type == "post"]{title, slug, body, mainImage}`
2const posts = await client.fetch(query)
Use getStaticProps in pages/index.js:
1export async function getStaticProps() {
2 const posts = await client.fetch(`*[_type == "post"]`)
3 return { props: { posts } }
4}
5. ✅ Render Content with Portable Text
Install:
1npm install @portabletext/react
1import { PortableText } from '@portabletext/react'
2
3<PortableText value={post.body} />
6. ✅ Handle Images
1import imageUrlBuilder from '@sanity/image-url'
2
3const builder = imageUrlBuilder(client)
4const urlFor = (source) => builder.image(source)
Usage:
1<img src={urlFor(post.mainImage).width(600).url()} alt="Post image" />
7. ✅ Setup Dynamic Routing (e.g., [slug].js)
1export async function getStaticPaths() {
2 const posts = await client.fetch(`*[_type == "post"]{slug}`)
3 return {
4 paths: posts.map((post) => ({ params: { slug: post.slug.current } })),
5 fallback: 'blocking',
6 }
7}
8. ✅ Enable Preview Mode (Optional but Recommended)
Sanity supports live preview of draft content. You can configure preview mode using environment variables and secure preview tokens.
🎯 Real-World Use Cases
- Blogs with real-time content updates
- Marketing websites
- Portfolios with dynamic CMS control
- Multi-language websites
🧩 Tips for Production
- Enable image optimization with Next.js
- Use ISR for frequent content updates
- Secure API tokens via .env.local
- Use Sanity’s webhooks to trigger revalidation
📈 Conclusion
Combining Next.js and Sanity.io gives you an unmatched combination of performance, flexibility, and developer experience. Whether you're building a blog, e-commerce site, or marketing platform, this duo sets you up for long-term success.
Want to build modern, scalable, and content-rich web applications? Start integrating Sanity with Next.js today!